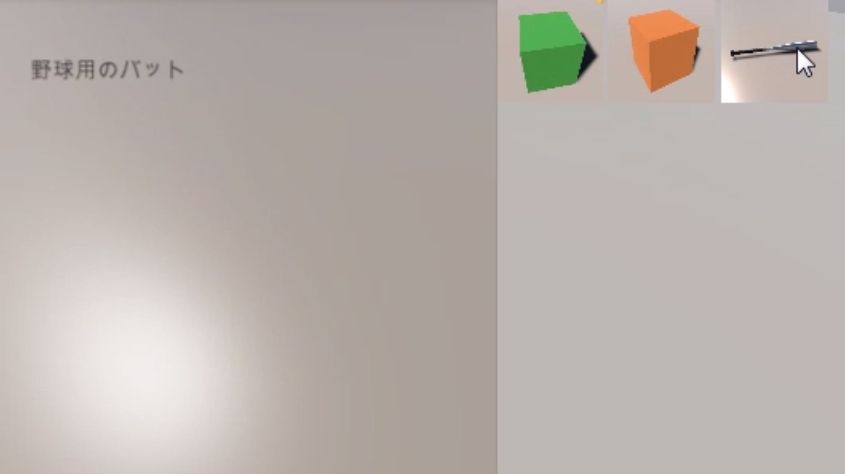
カーソルと重なっているUIのオブジェクトを取得して、インベントリのアイテムの情報を表示してみました。
メニュー表示を切り替えるスクリプトに、UIオブジェクトを取得する処理を追加しました。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.EventSystems;
class DisplayMenuOperation : DisplayMenu
{
bool opened;
PointerEventData pointer;
[SerializeField] Text text;
void Start()
{
pointer = new PointerEventData(EventSystem.current);
}
// Update is called once per frame
void Update()
{
if (Input.GetKeyDown(KeyCode.G))
{
if (GetMenuActiveSelf(0))
{
CloseMenu(0);
opened = false;
}
else
{
CloseAllCanvas();
OpenMenu(0);
opened = true;
}
}
else if (Input.GetKeyDown(KeyCode.Alpha2))
{
if (GetMenuActiveSelf(1))
{
CloseMenu(1);
opened = false;
}
else
{
CloseAllCanvas();
OpenMenu(1);
opened = true;
}
}
text.text = "";
if (opened)
{
List<RaycastResult> results = new List<RaycastResult>();
pointer.position = Input.mousePosition;
EventSystem.current.RaycastAll(pointer, results);
foreach (RaycastResult target in results)
{
Goods goods = target.gameObject.GetComponent<Goods>();
if (goods != null)
{
text.text = ItemManager.GetInstance().GetItem(goods.GetItemName()).GetInformation();
}
//text.text += target.gameObject.name + "\n";
}
}
}
}
メニューが開いているときだけ、イベントシステムでカーソルと重なっているUIのゲームオブジェクトを取得します。
名前を表示してみると、はじめはスクロールビューやViewportは取得できますが、アイテムのImageオブジェクトは無視されています。
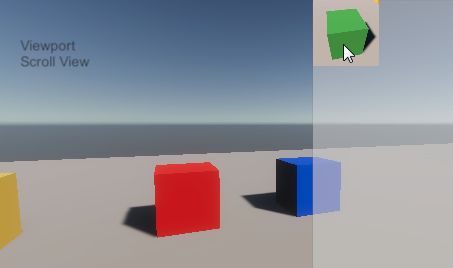
ここで取得できるのはImageコンポーネントのRaycast Targetのチェックが入っているものだけです。Scroll ViewやViewport、Scrollbarはもともとチェックが入っていました。
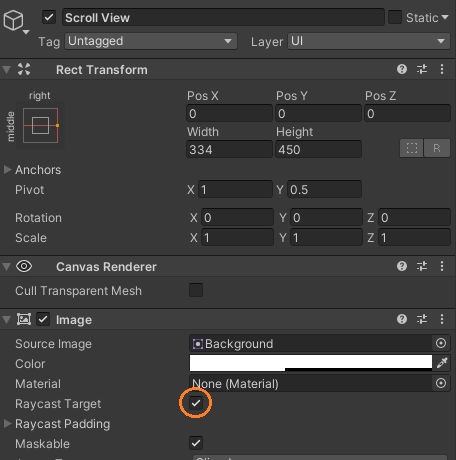
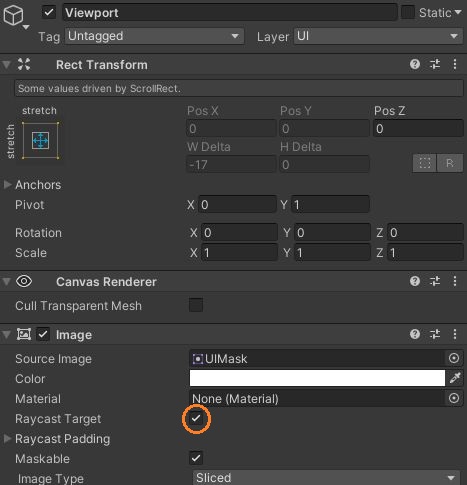
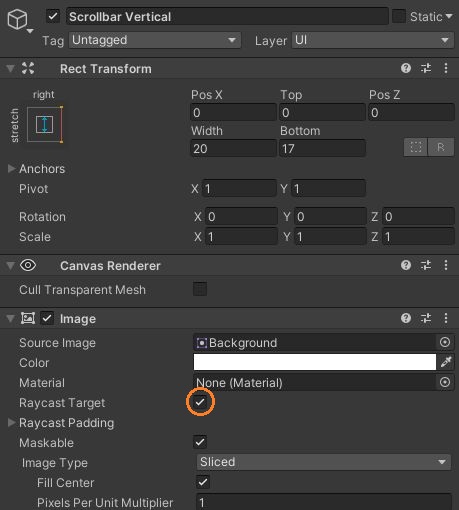
しかしアイテム画像のプレハブでは、チェックが入っていないので、このチェックを入れて他は切ります。
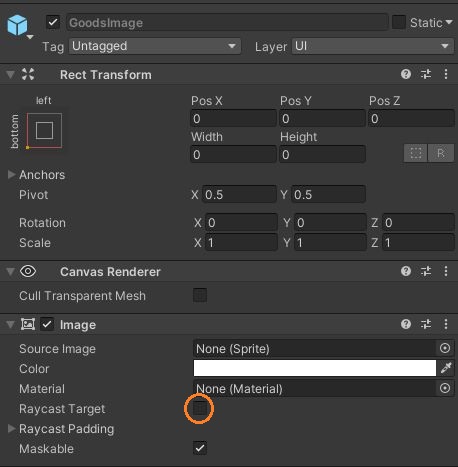
するとアイテムのImageオブジェクトだけが取得できました。
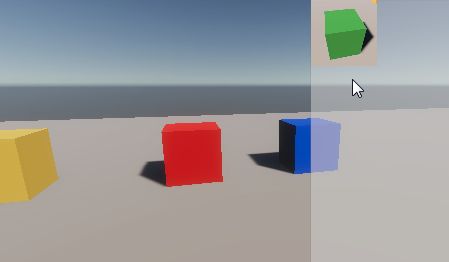
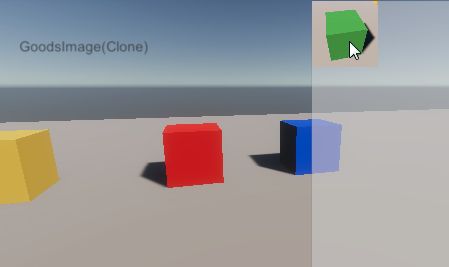
このプレハブには、アイテムを拾って管理するでアイテムの情報を保持するスクリプトを付けたので、それを取得しています。
そして、アイテム管理クラスのメソッドを引数にアイテム名を入れて呼ぶことで、アイテムのScriptableObjectが持つアイテムの情報を取得して表示しました。
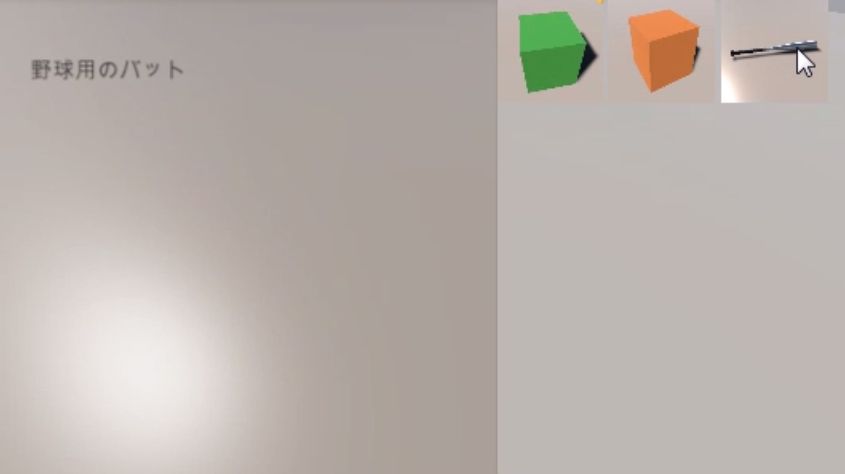
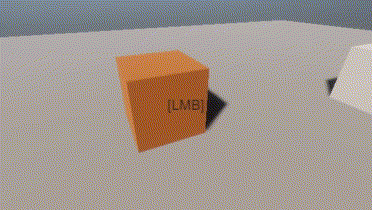
これで必要なUIオブジェクトだけを取得して、インベントリのアイテムの操作ができました。