敵キャラなどに3D表示の体力ゲージを付けてみます。
UIのImageオブジェクトをシーンに新規作成して、CanvasのRender Modeを「World Space」にすると3D表示になります。
CanvasとImageをAIキャラクターの子オブジェクトにしました。
Canvasのポジションを全て0にします。
Imageのポジションも0にして、シーンを見ながらWidthとHeightを変えて細長くします。
Canvasを真上に持ち上げて、キャラクターの頭上に置きました。
3D表示になっています。
Imageコンポーネントに白い画像を付けて、表示方式を変えます。ゲージの色を変えるためのスクリプトも付けました。
Imageでゲージを作る方法
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ImageScript : MonoBehaviour
{
Image image;
// Start is called before the first frame update
void Start()
{
image = GetComponent<Image>();
}
// Update is called once per frame
void Update()
{
if (image.fillAmount > 0.5f)
{
image.color = Color.green;
}
else if (image.fillAmount > 0.2f)
{
image.color = new Color(1f, 0.67f, 0f);
}
else {
image.color = Color.red;
}
}
}
新しく空のゲームオブジェクトを作って、AIキャラクターに目的地を教えたり、ゲージを増減させたりします。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
using UnityEngine.UI;
public class GameScript1 : MonoBehaviour
{
[SerializeField]
NavMeshAgent agent;
[SerializeField]
Transform[] points;
int n = 1;
[SerializeField]
Image image;
// Start is called before the first frame update
void Start()
{
agent.destination = points[0].position;
}
// Update is called once per frame
void Update()
{
// エージェントに目的地を設定
if (!agent.pathPending && agent.remainingDistance < 0.5f)
{
agent.destination = points[n].position;
n++;
if (n > 3) n = 0;
}
// ゲージを増減
if (Input.GetMouseButton(0))
{
if (image.fillAmount > 0f)
{
image.fillAmount -= Time.deltaTime;
}
else
{
image.fillAmount = 0f;
}
}
else {
if (image.fillAmount < 1f)
{
image.fillAmount += Time.deltaTime;
}
else
{
image.fillAmount = 1f;
}
}
}
}
これで敵キャラクターの体力ゲージなどをスクリプトで増減できますが、キャラクターに合わせてゲージの向きが変わるとゲージが見にくいです。
常にカメラの方を向かせる
Canvasにスクリプトを付けました。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RotateCanvas : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
transform.LookAt(Camera.main.transform.position);
}
}
LootAtメソッドを使って、常にメインカメラの方を向かせます。ゲージの表がCanvasの正面(Z軸方向)を向いているので、これがカメラを向けば、常にカメラからゲージが見えます。
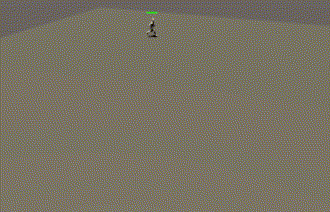
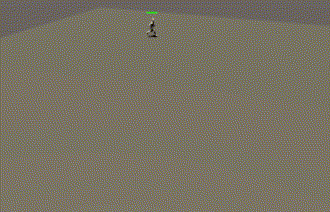
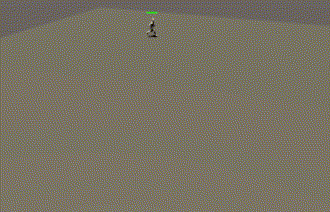